Introduction
In salesforce development, a Toast Message is a small, non-modal message that appears on the screen to notify the user of a specific event or action. These messages are typically used to provide feedback on a user’s actions, such as a successful login or a form submission.
Toast Messages are often used in mobile apps and are becoming increasingly popular in web applications as well.
With LWC, developers can create custom Toast Messages that can be easily integrated into their applications, providing a seamless user experience.
Creating a Toast Message in LWC
The following is a step-by-step guide to creating a Toast Message in LWC:
Step 1
Create a new Lightning Web Component (LWC) by following the steps outlined in the Salesforce documentation.
Step 2
In the HTML file of your new LWC, add the following code to create a button that will trigger the Toast Message:
<lightning-button label="Show Toast" onclick={showToast}></lightning-button>
Step 3
In the JavaScript file of your LWC, add the following code to create the Toast Message:
import { LightningElement, track } from 'lwc';
import { ShowToastEvent } from 'lightning/platformShowToastEvent';
export default class ToastExample extends LightningElement {
showToast() {
const event = new ShowToastEvent({
title: 'Success',
message: 'The Toast Message has been shown.',
variant: 'success',
});
this.dispatchEvent(event);
}
}
Step 4
Save your changes and test your new Toast Message by clicking on the button you created in step 2.
The code above creates a Toast Message with the title “Success” and the message “The Toast Message has been shown.” The variant attribute is set to “success”, which will display the Toast Message with a green background.
Complete Code examples:
import { LightningElement, track } from 'lwc';
import { ShowToastEvent } from 'lightning/platformShowToastEvent';
export default class ToastExample extends LightningElement {
showToast() {
const event = new ShowToastEvent({
title: 'Success',
message: 'The Toast Message has been shown.',
variant: 'success',
});
this.dispatchEvent(event);
}
}
<template>
<lightning-button label="Show Toast" onclick={showToast}></lightning-button>
</template>
Customizing Toast Messages in LWC
Toast Messages in LWC can be customized to match the look and feel of your application. Some of the ways to customize Toast Messages include:
Changing the appearance: You can change the background color, text color, and font of Toast Messages by using the variant attribute. The variant attribute can be set to “success”, “warning”, “error”, “info”, or “offline”.
Adding icons and images: You can add icons or images to Toast Messages by using the iconName attribute. The iconName attribute can be set to any of the icons available in the Lightning Design System.
Creating custom templates: You can create custom templates for Toast Messages by using the template attribute. This attribute can be set to a custom Lightning Web Component that you have created.
Toast Message for Success
//html
<template>
<lightning-button label="Show success message" onclick={showToastForSuccess}></lightning-button>
// Js
import { LightningElement } from 'lwc';
import { ShowToastEvent } from 'lightning/platformShowToastEvent';
showToastForSuccess() {
const evt = new ShowToastEvent({
title: 'save',
message: 'event success!',
variant: 'success',
});
this.dispatchEvent(evt);
}
Output
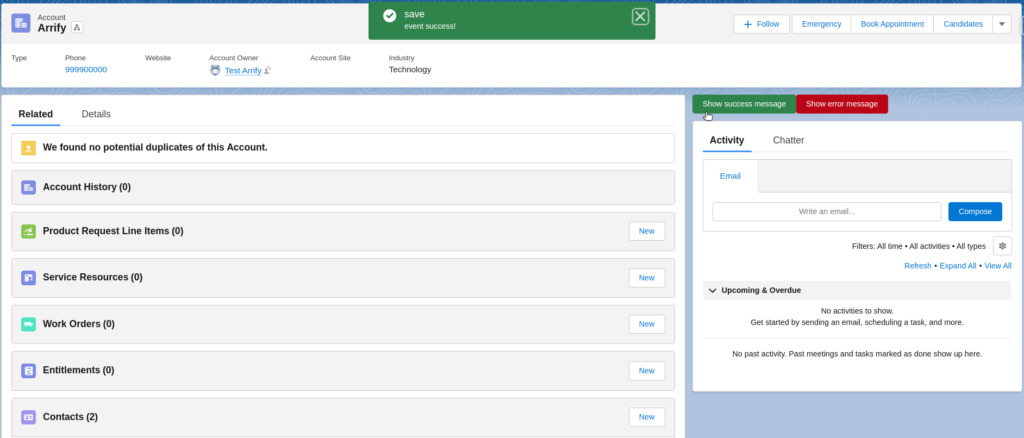
Toast Message for Error
//html
<template>
<lightning-button label="Show error message" onclick={showToastForError}></lightning-button>
</template>
// Js
import { LightningElement } from 'lwc';
import { ShowToastEvent } from 'lightning/platformShowToastEvent';
showToastForError() {
const evt = new ShowToastEvent({
title: 'error',
message: 'Error Occur',
variant: 'error',
});
this.dispatchEvent(evt);
}
Output
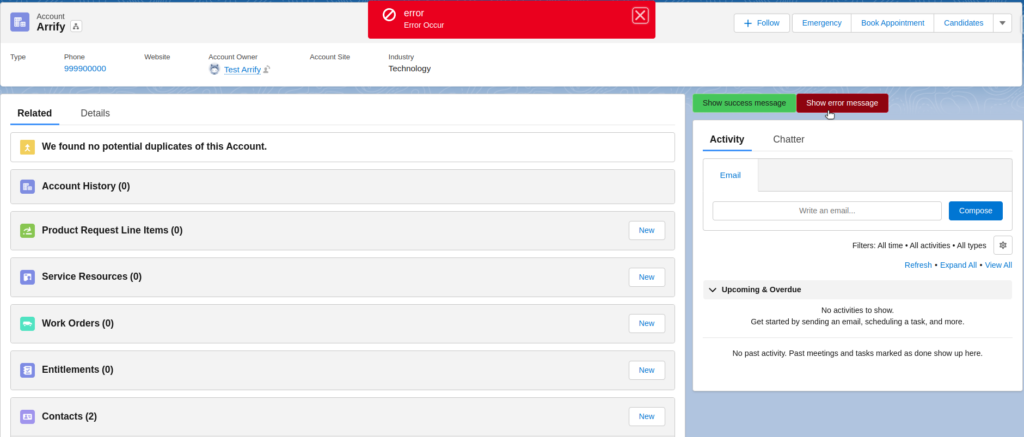
Toast Message for Information
//html
<template>
<lightning-button variant="destructive" label="Show info message" onclick={showToastForInfo}></lightning-button>
</template>
// Js
import { LightningElement } from 'lwc';
import { ShowToastEvent } from 'lightning/platformShowToastEvent';
showToastForInfo() {
const evt = new ShowToastEvent({
title: 'information',
message: 'event information',
variant: 'info',
});
this.dispatchEvent(evt);
}
Output
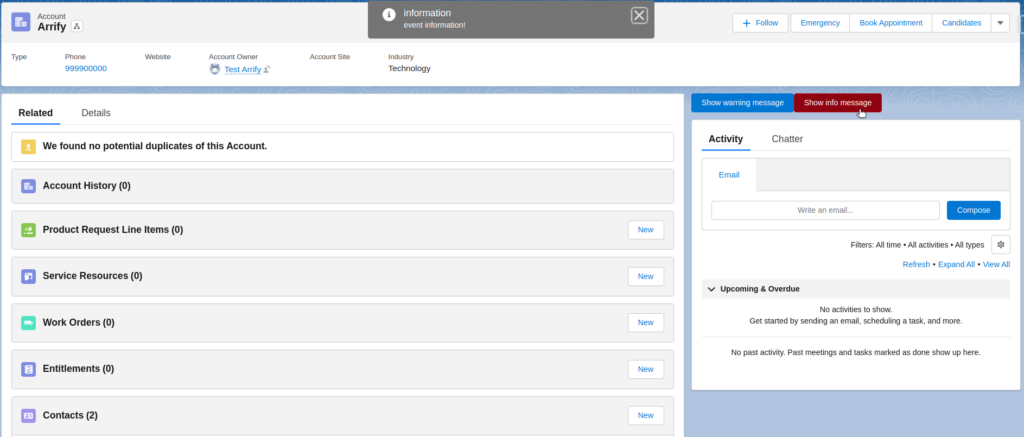
Toast Message for Warning
//html
<template>
<lightning-button variant="brand" label="Show warning message" onclick={showToastForWarning}></lightning-button>
</template>
// Js
import { LightningElement } from 'lwc';
import { ShowToastEvent } from 'lightning/platformShowToastEvent';
showToastForWarning() {
const evt = new ShowToastEvent({
title: 'warning',
message: 'event warning',
variant: 'warning',
});
this.dispatchEvent(evt);
}
Output
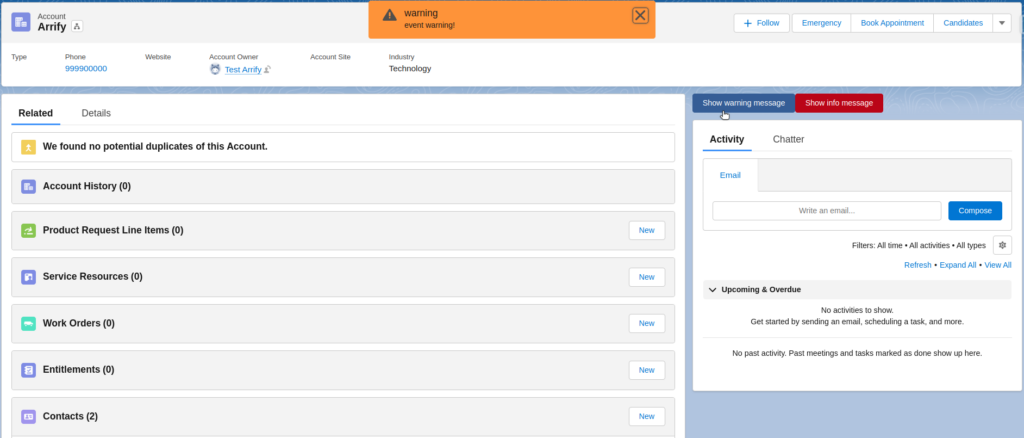
Best Practices for Toast Messages in LWC
When using Toast Messages in LWC, it’s important to follow best practices to ensure that they are effective and user-friendly. Some best practices to keep in mind include:
- Keep messages brief and to the point: Toast Messages should be short and convey a specific message. Avoid using long sentences or complex language.
- Use appropriate colors and icons: Use colors and icons that match the message and the overall design of your application. For example, use a red background for error messages and a green background for success messages.
- Limit the number of Toast Messages: Too many Toast Messages can be overwhelming for users and can make it difficult for them to focus on the task at hand. Limit the number of Toast Messages that appear on the screen at one time.
- Use Toast Messages to provide feedback, not instructions: Toast Messages should be used to provide feedback on a user’s actions, not to provide instructions on how to use the application.
- Test your Toast Messages: Before deploying your application, test your Toast Messages to ensure that they are working as expected and that they are easy to understand.
By following these best practices, developers can create Toast Messages in LWC that are effective, user-friendly, and provide valuable feedback to users of the Salesforce platform.
Conclusion
By following best practices and customizing Toast Messages, developers can create Toast Messages that are effective, user-friendly and provide valuable feedback to users of the Salesforce platform.
To learn more about Toast Messages in LWC and other features of the Salesforce platform, visit the Salesforce Developer website and the Lightning Web Components Developer Guide. These resources provide detailed information and tutorials on how to create, customize and implement Toast Messages in LWC.
https://www.reddit.com/r/Salesforcew3web/comments/r48r2k/how_to_display_toast_message_with_hyperlink_and/: Toast Message in LWC
Leave a Reply