Introduction to LWC DataTable
Ah, Lightning Data Tables – sounds electrifying, doesn’t it? But if you’re wondering what it really means, you’re in the right spot.
What Exactly is a Lightning Data Table?
Lightning Data Table is a Salesforce component that provides a flexible and customizable table layout for displaying lists of information. Think of it as a nifty tool that lets you showcase data in a neat, organized format without the headache of starting from scratch. You get sortable columns, inline editing, and even pagination. Basically, it’s like getting a Swiss Army knife for your data presentation needs.
Advantages of Using It in LWC (Lightning Web Components)
Now, coupling Lightning Data Tables with LWC is where the real magic happens. Here’s why:
Speed and Efficiency
LWC’s modern architecture means faster rendering and a smoother user experience.
Flexibility
You can customize your tables to the moon and back, tailoring them to fit your unique requirements.
Interactivity
With LWC, you get event handling, making your tables not just static displays but interactive elements.
Consistency
Using standard components ensures a unified look and feel, aligning with Salesforce’s design philosophy.
Setting the Stage
Before we dive deep, there are a few things you’ll need to set the stage. Think of this as gathering your ingredients before whipping up a gourmet dish.
Prerequisites and Things You Need to Know:
Salesforce Org
You’ll need a Salesforce org. If you don’t have one, you can sign up for a free Developer Edition (DE) org.
Dev Console or an IDE
This is where you’ll be doing the coding magic. Salesforce Developer Console is a good starting point, but if you’re feeling fancy, Salesforce extensions for Visual Studio Code is a top pick.
Basic Knowledge of LWC
While I’ll walk you through the steps, having a basic understanding of LWC will make the journey smoother.
Apex
Since we’ll be fetching data from Salesforce, knowing a bit about Apex, Salesforce’s programming language, is beneficial.
A Good Cup of Coffee (or Tea)
Optional, but hey, a little caffeine never hurt anyone, right?
Now, with our checklist ready, let’s jump into the actual building process!
Building the Lightning Web Component (LWC)
Creating a Lightning Web Component (LWC) is akin to crafting a miniature masterpiece. We’ll commence with the structure, build up the functionality, and polish off with some style.
The Framework : LwcDataTableExample.html
Your HTML file is your foundation. This is where you define the skeleton of your data table.
<template>
<lightning-card title="LWC data-table Demo">
<lightning-datatable
data= {data}
columns={columnsArray}
key-field="Id"
onrowselection={handleRowSelection}
sorted-by={sortBy}
onsort={doSorting}
sorted-direction= {sortDirection}>
</lightning-datatable>
<div class="slds-p-around_medium">
<lightning-layout class="vertical-align">
<lightning-layout-item>
<lightning-button label="Previous" icon-name="utility:chevronleft" onclick={previousPage}></lightning-button>
</lightning-layout-item>
<lightning-layout-item>
Page {page} of {totalPage}
</lightning-layout-item>
<lightning-layout-item>
<lightning-button label="Next" icon-name="utility:chevronright" icon-position="right" onclick={nextPage}></lightning-button>
</lightning-layout-item>
</lightning-layout>
</div>
</lightning-card>
</template>
Bringing It to Life : LwcDataTableExample.js
JavaScript powers the interactivity and dynamic behaviors of your LWC. This .js
file is where all the action happens.
import { LightningElement,api,wire, track} from 'lwc';
import fetchObjectData from '@salesforce/apex/LwcDataTableExampleController.fetchObjectData';
export default class LwcDataTableExample extends LightningElement {
@track sObjectApiName = 'Account'; // Object API name
@track sObjectFieldsApiName = 'Name,Phone,Industry'; // Object's fields API name
@track sortBy;
@track sortDirection;
@track page = 1; //Initialize starting page
@track items = []; // Get record based on page selection
@track data = []; // Store return data
@track startingRecord = 1; //First record of table to display
@track endingRecord = 0; // Last record of table to display
@track pageSize = 10; //Display record per page
@track totalRecountCount = 0; //Total length of records
@track totalPage = 0; //Initially total page 0, it will be evaluate by total length of records
@track isPageChanged = false; //Track page when it change
//Split sObjectFieldsApiName with comma and add into list
get objFieldsArray() {
return this.sObjectFieldsApiName.split(',');
}
//Generate table columns dynamically
get columnsArray() {
return this.objFieldsArray.map(fieldName => {
return {
label: fieldName,
fieldName: fieldName,
type: 'text',
sortable: true,
};
});
}
//Get object records
@wire(fetchObjectData, { objectApiName: '$sObjectApiName' , fieldApiNames : '$sObjectFieldsApiName'})
searchResult(value) {
const { data, error } = value;
if (data) {
console.log('data');
console.log(JSON.parse(JSON.stringify(data)));
this.processRecords(data);
}
else if (error) {
console.log('(error---> ' + error);
}
};
// This method process data after fetching data
processRecords(data){
this.items = data;
this.totalRecountCount = data.length;
this.totalPage = Math.ceil(this.totalRecountCount / this.pageSize);
this.data = this.items.slice(0,this.pageSize);
this.endingRecord = this.pageSize;
}
//Method invoke on previous button click
previousPage() {
this.isPageChanged = true;
if (this.page > 1) {
this.page = this.page - 1;
this.displayRecordPerPage(this.page);
}
}
//Method invoke on next button click
nextPage() {
this.isPageChanged = true;
if((this.page<this.totalPage) && this.page !== this.totalPage){
this.page = this.page + 1;
this.displayRecordPerPage(this.page);
}
}
//This method display record by per page based on page selection
displayRecordPerPage(page){
this.startingRecord = ((page -1) * this.pageSize) ;
this.endingRecord = (this.pageSize * page);
this.endingRecord = (this.endingRecord > this.totalRecountCount)
? this.totalRecountCount : this.endingRecord;
this.data = this.items.slice(this.startingRecord, this.endingRecord);
this.startingRecord = this.startingRecord + 1;
}
//Method invoke on row selection
handleRowSelection(event) {
const selectedRows = event.detail.selectedRows;
if (selectedRows.length > 0) {
console.log('selected row');
console.log(selectedRows);
// Show an alert when rows are selected
alert('Selected Records : ' + selectedRows.map(row => row.Name).join(', '));
}
}
//Process sorting
doSorting(event) {
this.sortBy = event.detail.fieldName;
this.sortDirection = event.detail.sortDirection;
this.sortData(this.sortBy, this.sortDirection);
}
//This method sort data
sortData(fieldName, direction) {
let parseData = {};
parseData = JSON.parse(JSON.stringify(this.data));
let keyValue = (a) => {
return a[fieldName];
};
let isReverse = direction === 'asc' ? 1: -1;
parseData.sort((x, y) => {
let xVal = keyValue(x) ? keyValue(x) : ''; // handling null values
let yVal = keyValue(y) ? keyValue(y) : '';
// sorting values based on direction
return isReverse * ((xVal > yVal) - (yVal > xVal));
});
this.data = parseData;
}
}
Making It Look Good : LwcDataTableExample.css
Your data table needs some aesthetic finesse. The CSS lends a visual touch to make the table appealing.
.vertical-align {
align-items: center;
justify-content: space-between;
}
The Essentials : LwcDataTableExample.html Metadata
This is the administrative segment of your component, determining its behavior and integration within Salesforce.
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>58.0</apiVersion>
<description>Lwc Data Table Example</description>
<isExposed>true</isExposed>
<masterLabel>Lwc Data Table Example</masterLabel>
<targets>
<target>lightning__AppPage</target>
<target>lightning__HomePage</target>
<target>lightning__RecordPage</target>
</targets>
</LightningComponentBundle>
Diving into the Apex Class
The Apex Class is the vital link between your LWC and the Salesforce data troves.
An Overview of LwcDataTableExampleController
The LwcDataTableExampleController
class acts as the orchestrator, ensuring your data is fetched and processed correctly.
public class LwcDataTableExampleController {
@AuraEnabled(Cacheable=true)
public static List<SObject> fetchObjectData(String objectApiName, String fieldApiNames) {
String query = 'SELECT ' + fieldApiNames + ' FROM ' + objectApiName;
return Database.query(query);
}
}
Remember, your Apex class is vital. Treat it as the backbone that supports and feeds your Lightning Web Component with the data it needs to shine.
Output – Seeing Your Creation in Action
After the diligent work in setting up your LWC, it’s showtime! Displaying the output ensures that everything we’ve crafted so far comes together in harmony.
Visual Display
Your Lightning Data Table will manifest itself within the Salesforce interface as a well-structured table. The data is neatly presented in rows and columns, with sorting capabilities lending users a refined experience.
The pagination at the bottom enables navigation through data sets with ease. The “Previous” and “Next” buttons ensure smooth transitions between pages, while the page number indicator gives clarity on positioning within the data set.
Functionality Check
Interact with your table. Here’s what you should observe:
Sorting
Clicking on a column header toggles between ascending and descending sorts.
Row Selection
Clicking on a row triggers the handleRowSelection
function, which, for our current setup, displays an alert with details of the selected records.
Pagination
Navigating with the “Previous” and “Next” buttons updates the data display according to the selected page, and the page counter reflects the current page number.
Fine-tuning and Best Practices
Creating is one thing, optimizing is another. Let’s ensure our Lightning Web Component isn’t just functional, but also robust and efficient.
Error Handling
Always anticipate the unexpected. Ensure that your Apex method and LWC JavaScript have error handling mechanisms in place. This is crucial to gracefully handle any unforeseen circumstances or data hiccups.
Optimize Queries
Your current Apex query fetches data directly. While this is fine for our demonstration, remember that in real-world scenarios, it’s essential to add WHERE clauses, LIMIT statements, and possibly even ORDER BY clauses to refine the data retrieval.
Modular Code
Your JavaScript code for the LWC is rich and functional. However, consider breaking down larger methods into smaller, more focused functions. This not only enhances readability but also makes maintenance easier.
CSS Consistency
If you’re building multiple components or working within a team, ensure that your CSS naming conventions and styles remain consistent. It helps in creating a unified look and feel across all components.
Testing
Never underestimate the power of testing. Before deploying your LWC to a production environment, subject it to rigorous testing—both functional and user acceptance—to ensure it meets business needs and is free from bugs.
To sum up, while the construction of your Lightning Web Component is an achievement in itself, the real magic lies in refining, testing, and ensuring it’s optimized for the best user experience. Happy coding!
Output
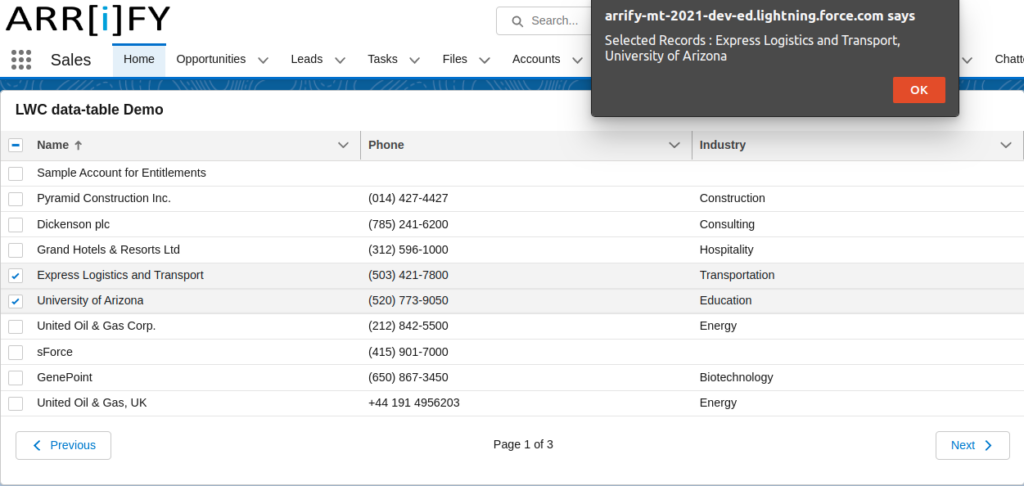
Conclusion – Wrapping it All Up
We’ve embarked on quite a journey, haven’t we? From the initial stages of creating a Lightning Web Component to viewing its output, fine-tuning it, and learning best practices along the way, we’ve covered substantial ground. It’s essential to not only understand the technicalities but also to appreciate the broader picture of why and how these components make a difference in the Salesforce ecosystem.
Key Takeaways
Lightning Data Tables
It offer a structured and interactive way to display data within the Salesforce platform. By leveraging their capabilities, developers can provide end-users with a powerful tool to sift through data with ease.
Flexibility & Customization
One of the significant advantages of LWC is its adaptability. Whether it’s the data displayed, the functionalities offered, or the look and feel, everything can be tailor-made to fit exact requirements.
Coding Best Practices
As we saw, while creating a functioning component is a start, adhering to best practices ensures maintainability, efficiency, and overall robustness. Always focus on writing clean, modular, and optimized code.
Continuous Learning
Salesforce and its suite of tools are ever-evolving. It’s crucial to keep updated with the latest trends, tools, and best practices in the Salesforce world. This not only helps in building better components but also offers a competitive edge in the market.
Final Thoughts
Building a Lightning Web Component, like the data-table we discussed, is a blend of technical know-how, understanding user requirements, and an overarching vision to improve the Salesforce user experience. Always approach LWC creation with a problem-solving mindset, seeking to address specific challenges or improve existing processes.
Thank you for joining me on this journey through Lightning Web Components. I hope this deep dive provides you with a strong foundation and the confidence to create, optimize, and deploy powerful components within Salesforce. Until next time, keep innovating and happy coding!
Frequently Asked Questions (FAQ)
What is a Lightning Data Table in Lightning Web Components?
Lightning Data Table is a component provided by Salesforce to display tabular data with interactive features such as sorting, row selection, and inline editing. It’s a powerful tool for developers to present data in a structured format without building a table from scratch.
Can I customize the appearance of my Lightning Data Table?
Yes, you can customize the appearance using CSS. Additionally, you can define the columns in the JavaScript, allowing for a more flexible representation of data fields.
How do I handle large data sets with Lightning Data Tables?
For large data sets, consider implementing pagination, as demonstrated in the tutorial. It allows users to navigate through data sets smoothly without overwhelming the browser or the system with excessive data at once.
Can the Lightning Data Table connect to external data sources or only Salesforce objects?
The example provided demonstrates pulling data from Salesforce objects. However, with additional development, you can connect to external data sources using Apex callouts or other integrative techniques.
Is inline editing supported in Lightning Data Tables?
Yes, Lightning Data Tables support inline editing. By setting the editable attribute for specific columns, users can modify the data directly within the table.
How do I refresh the Lightning Data Table after making data changes?
You can utilize the refreshApex function provided by Salesforce to refresh wired data after making changes, ensuring the table displays the most recent data.